Migrating Legacy Code from Class Components to Functional Components in React
Introduction
React functional components, introduced with the introduction of hooks, have become the recommended approach for building components in React. However, many existing React projects still use class components. This blog post will guide you through the process of migrating legacy code from class components to functional components. We will discuss the benefits of using functional components, the step-by-step migration process, and provide practical examples and tips to make the transition smoother.
Benefits of Functional Components
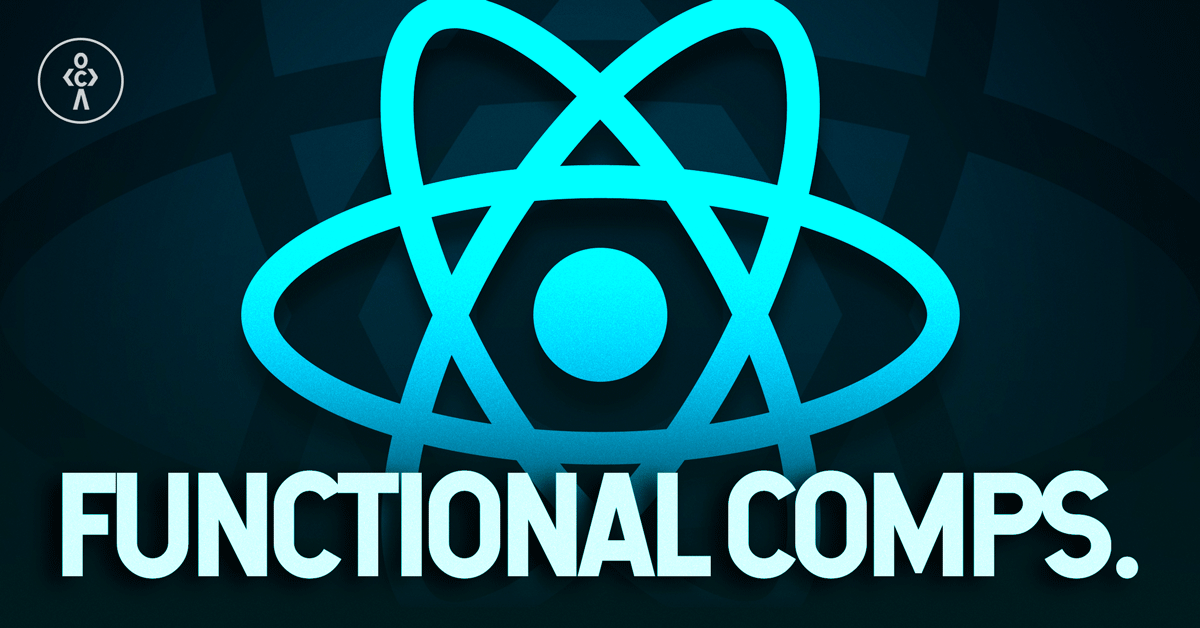
Pic Courtesy: Codedamn
- Simpler Syntax: Functional components use JavaScript functions, resulting in a cleaner and more concise syntax compared to class components.
- Improved Performance: Functional components can leverage React hooks to optimize performance, enable better code splitting, and reduce the number of re-renders.
- Enhanced Readability: Functional components promote easier comprehension and code readability by focusing on the core logic and reducing boilerplate code.
- Encourages Composition: Functional components encourage the use of composition and functional programming principles, making code organization and reuse more straightforward.
Migrating Class Components to Functional Components
To migrate class components to functional components, follow these step-by-step instructions:
Step 1: Identify the Class Component to Migrate
Identify the class component you want to migrate to a functional component. Understand its functionality, state, lifecycle methods, and any dependencies on external libraries.
Step 2: Rewrite the Class Component as a Function
Rewrite the class component as a functional component by creating a new JavaScript function. Move the component's
logic and state management into the function body. Remove lifecycle methods and replace them with equivalent hooks,
such as useState
, useEffect
, or custom hooks.
Step 3: Update the Component Usage and Dependencies
Update the usage of the component throughout your project, replacing the old class component with the new functional component. Ensure that any external library dependencies are handled appropriately and that the component integrates seamlessly with other parts of the application.
Example Migration
Let's consider a practical example of migrating a class-based counter component to a functional component using hooks:
import React, { Component } from 'react';
class Counter extends Component {
constructor(props) {
super(props);
this.state = {
count: 0
};
}
componentDidMount() {
// Component initialization logic
}
componentDidUpdate(prevProps, prevState) {
// Component update logic
}
componentWillUnmount() {
// Component cleanup logic
}
incrementCount() {
this.setState({ count: this.state.count + 1 });
}
render() {
return (
<div>
Count: {this.state.count}
<button onClick={() => this.incrementCount()}>Increment</button>
</div>
);
}
}
export default Counter;
import React, { useState, useEffect } from 'react';
const Counter = () => {
const [count, setCount] = useState(0);
useEffect(() => {
// Component initialization and update logic
return () => {
// Component cleanup logic
};
}, []);
const incrementCount = () => {
setCount(count + 1);
};
return (
<div>
Count: {count}
<button onClick={incrementCount}>Increment</button>
</div>
);
};
export default Counter;
Conclusion
Migrating legacy code from class components to functional components in React offers numerous benefits, including a simplified syntax, improved performance, enhanced readability, and encouragement of composition. By following the step-by-step migration process, you can seamlessly transition your codebase to leverage the power and advantages of functional components. Remember to thoroughly test your migrated components and ensure proper integration with the existing codebase. With careful planning and execution, the migration process can lead to a more efficient and maintainable React application.
Comments
Post a Comment